How to learn JavaScript : JavaScript Tutorial for Beginners step by step
Table of Contents
1. What is JavaScript?
JavaScript is a scripting language that enables you to add functionality to web pages. It’s an integral part of web development, making your sites more engaging and responsive.
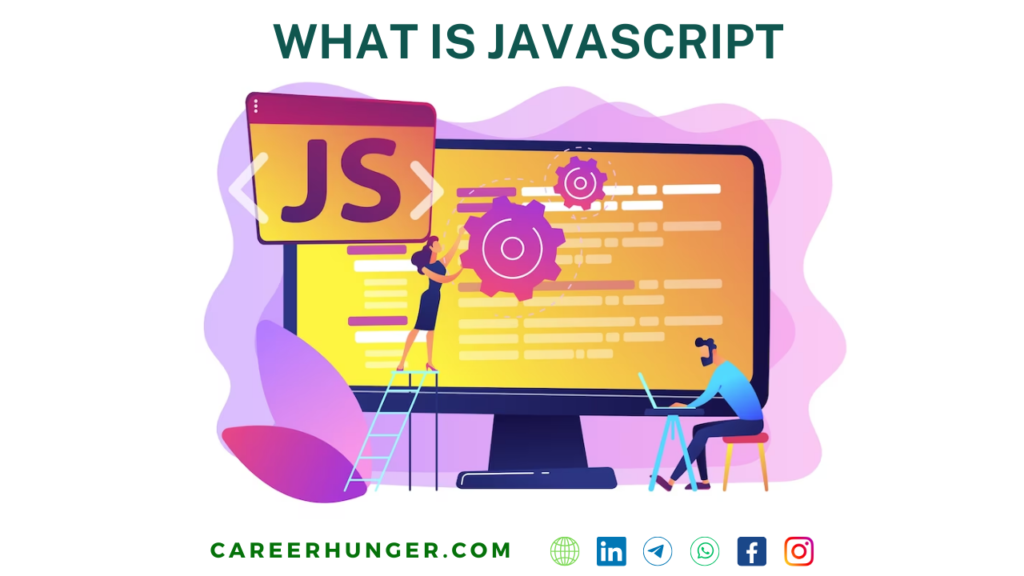
JavaScript First Steps
Welcome to the exciting world of JavaScript! Whether you’re a complete novice or have dabbled in programming before, learning JavaScript opens up a myriad of opportunities in web development. In this comprehensive guide, we’ll take you through the essential concepts of JavaScript, from its basic syntax to more advanced topics like asynchronous programming and working with APIs. By the end of this journey, you’ll have a solid understanding of JavaScript fundamentals and be well-equipped to build dynamic and interactive web applications. If you’re new to programming, you might be wondering, “What is JavaScript?” Well, JavaScript is a versatile programming language primarily used for web development. It allows you to make your websites interactive and dynamic. In this first module, we’ll answer some fundamental questions, provide an overview of JavaScript’s syntax, and guide you through your first practical experience of writing JavaScript code.
How to take input in JavaScript : JavaScript Syntax
Let’s take a look at what JavaScript code looks like. It consists of statements, which are executed line by line. Here’s a simple example:
// This is a comment
let greeting = "Hello, World!";
console.log(greeting);
In this snippet, we declare a variable greeting and assign it the value “Hello, World!”. We then use console.log() to output the greeting to the browser console.
Key JavaScript Features
Now, let’s delve into some key JavaScript features:
- Variables: Used to store and manipulate data.
- Strings: Sequences of characters, enclosed in single or double quotes.
- Numbers: Numeric data types for arithmetic operations.
- Arrays: Ordered lists of values.
Variables in JavaScript: Storing the Information
In JavaScript, variables are used to store information that can be referenced and manipulated in your code. You declare variables using the let, const, or var keywords.
let name = "John";
const age = 30;
var job = "Developer";
Basic math in JavaScript : numbers and operators in JavaScript
JavaScript supports various arithmetic operators for performing mathematical operations. JavaScript supports arithmetic operations like addition, subtraction, multiplication, and division. You can perform basic math operations using numeric values and operators.
let num1 = 10;
let num2 = 5;
let sum = num1 + num2;
let difference = num1 - num2;
let product = num1 * num2;
let quotient = num1 / num2;
Strings in JavaScript
Strings are sequences of characters enclosed in single or double quotes. They’re used to represent text data in JavaScript.
let greeting = "Hello, World!";
let message = 'Welcome to JavaScript!';
Useful string methods in JavaScript
JavaScript provides numerous built-in methods for manipulating strings. Some common string methods include toUpperCase(), toLowerCase(), charAt(), substring(), and split().
let text = "JavaScript is awesome";
console.log(text.toUpperCase()); // Outputs: JAVASCRIPT IS AWESOME
console.log(text.charAt(0)); // Outputs: J
console.log(text.substring(0, 10)); // Outputs: JavaScript
console.log(text.split(" ")); // Outputs: ["JavaScript", "is", "awesome"]
How to create array in JavaScript : Arrays
Arrays are used to store multiple values in a single variable. They are indexed collections of data. You can create arrays using square brackets and access elements by their index.
let fruits = ["apple", "banana", "orange"];
console.log(fruits[0]); // Outputs: apple
Arrays are used to store multiple values in a single variable. They are indexed collections of data. You can create arrays using square brackets and access elements by their index.
2. JavaScript Building Blocks
JavaScript Building Blocks are the fundamental elements that form the core of JavaScript programming. They provide the structure and functionality necessary to create robust and dynamic applications.
In this module, we’ll explore the fundamental building blocks of JavaScript. These include conditional statements, loops, functions, and events – crucial elements that form the backbone of any JavaScript program.
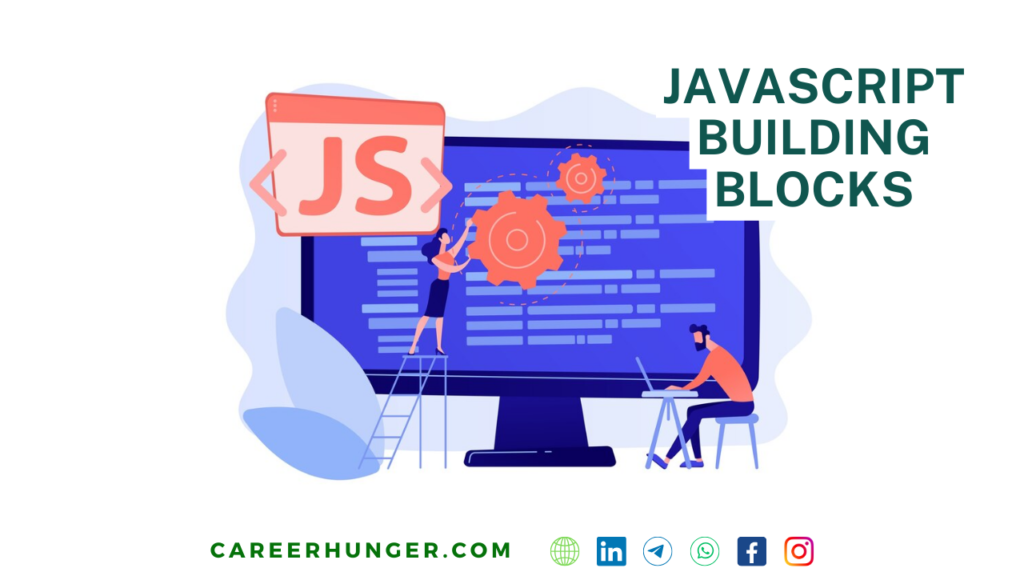
Conditional Statements in JavaScript
Conditional statements allow you to make decisions in your code based on certain conditions. These statements control the flow of execution by evaluating whether a condition is true or false. Common conditional statements include if, else if, and else. Here’s an example:
let age = 18;
if (age >= 18) {
console.log("You are eligible to vote!");
} else {
console.log("Sorry, you are too young to vote.");
}
Loops in JavaScript
Loops are used to execute a block of code repeatedly until a specified condition is met. They are handy for iterating over arrays, performing repetitive tasks, and controlling the flow of execution. JavaScript supports different types of loops, such as for, while, and do…while. Here’s an example of a for loop:
for (let i = 0; i < 5; i++) {
console.log(i);
}
How to create function in JavaScript: Functions
Functions are blocks of reusable code that perform a specific task. They allow you to modularize your code, improve readability, and avoid repetition. Functions can take input (parameters) and return output (result). Here’s a simple function that adds two numbers:
function add(a, b) {
return a + b;
}
let result = add(3, 5);
// result will be 8
What is event in JavaScript: Events
Events are actions or occurrences that happen in the browser, such as a user clicking a button or hovering over an element. You can use JavaScript to listen for these events and execute code in response. Event handling is crucial for creating interactive and dynamic web pages. Here’s an example of adding an event listener to a button:
document.getElementById("myButton").addEventListener("click", function() {
console.log("Button clicked!");
});
3. Introducing JavaScript Objects
JavaScript treats almost everything as objects. Understanding object-oriented programming is crucial. This module covers object theory, syntax, creating custom objects, and working with JSON data.
JavaScript Objects are a fundamental part of the language, allowing you to encapsulate data and functionality into reusable units. Let’s explore the various aspects of JavaScript objects in more detail:

JavaScript Object Basics
In JavaScript, objects are collections of key-value pairs, where each key is a string (or Symbol) and each value can be any data type. Objects are versatile and can represent complex entities with properties and methods. Here’s a simple example of creating and accessing object properties:
// Creating an object
let person = {
name: "John",
age: 30,
greet: function() {
console.log("Hello, " + this.name + "!");
}
};
// Accessing object properties and methods
console.log(person.name);
// John
person.greet();
// Hello, John!
Object Prototypes in JavaScript
In JavaScript, every object is linked to a prototype object from which it inherits properties and methods. Understanding prototypes is essential for working effectively with JavaScript objects and inheritance. Here’s a brief example:
// Creating a prototype
let animal = {
type: "Dog",
sound: "Woof!"
};
// Creating an object that inherits from the prototype
let myPet = Object.create(animal);
console.log(myPet.type);
// Dog
console.log(myPet.sound);
// Woof!
Object Oriented Programming in JavaScript
JavaScript supports object-oriented programming (OOP) paradigms, allowing you to create reusable and modular code by organizing data and behavior into objects. OOP concepts such as encapsulation, inheritance, and polymorphism are achievable in JavaScript. Here’s a simplified example of using OOP principles:
// Constructor function for creating objects
function Person(name, age) {
this.name = name;
this.age = age;
}
// Adding a method to the prototype
Person.prototype.greet = function() {
console.log("Hello, my name is " + this.name + "!");
};
// Creating an object using the constructor
let john = new Person("John", 30);
john.greet();
// Hello, my name is John!
How to add class in JavaScript : Classes in JavaScript
ES6 introduced class syntax to JavaScript, providing a more familiar and structured way to define objects and constructors. Classes act as blueprints for creating objects with predefined properties and methods. Here’s an example of using classes in JavaScript:
// Defining a class
class Car {
constructor(brand) {
this.brand = brand;
}
drive() {
console.log("Driving a " + this.brand);
}
}
// Creating an instance of the class
let myCar = new Car("Toyota");
myCar.drive();
// Driving a Toyota
What is JSON in JavaScript : JSON Data in JavaScript
JSON (JavaScript Object Notation) is a lightweight data interchange format commonly used for transmitting data between a server and a web application. JavaScript provides built-in methods for parsing and stringifying JSON data. Here’s an example:
// JSON data
let jsonString = '{"name": "John", "age": 30}';
// Parsing JSON
let data = JSON.parse(jsonString);
console.log(data.name);
// John
// Converting JavaScript object to JSON
let person = { name: "Alice", age: 25 };
let jsonData = JSON.stringify(person);
console.log(jsonData);
// {"name":"Alice","age":25}
4. Asynchronous JavaScript
This module explores the importance of asynchronous programming in JavaScript, especially for handling operations that may block execution, like fetching resources from a server.
Asynchronous JavaScript is a crucial aspect of modern web development, allowing you to execute non-blocking operations efficiently. Let’s explore the various aspects of asynchronous programming in JavaScript:
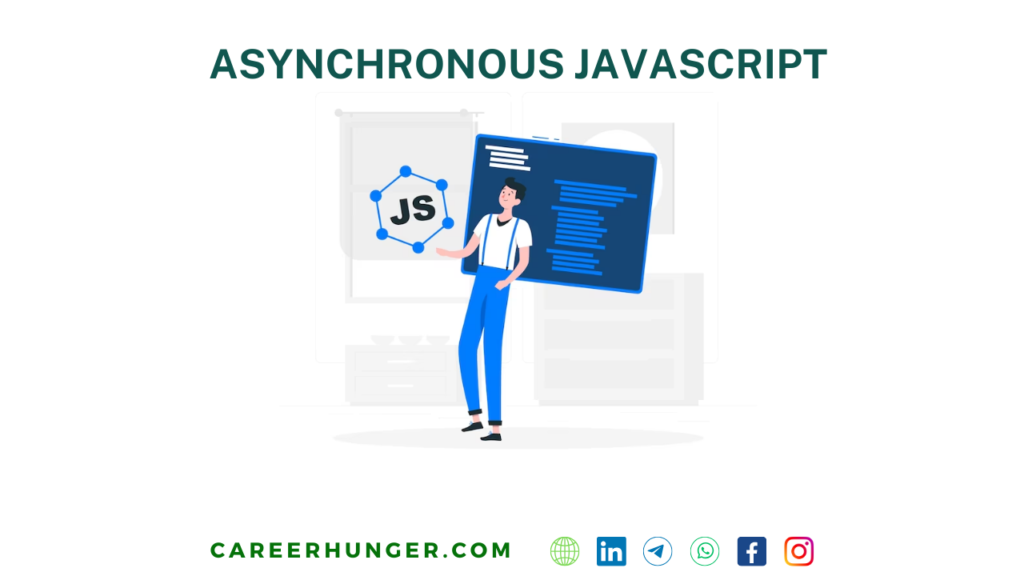
What is asynchronous in JavaScript
In traditional synchronous programming, code is executed sequentially, one statement at a time. Asynchronous JavaScript, on the other hand, enables you to perform operations without waiting for previous tasks to complete. This is particularly useful for tasks like fetching data from a server, handling user input, or performing time-consuming computations without blocking the main thread.
What is promise in JavaScript
Promises are a built-in JavaScript feature introduced in ES6, providing a cleaner and more structured way to handle asynchronous operations. A promise represents a value that may be available now, in the future, or never. Promises have three states: pending, fulfilled, or rejected. Here’s how you can create and use a promise:
// Creating a promise
let fetchData = new Promise((resolve, reject) => {
// Simulating an asynchronous operation
setTimeout(() => {
let data = { name: "John", age: 30 };
resolve(data); // Resolving the promise with data
}, 2000);
});
// Using the promise
fetchData.then((data) => {
console.log(data);
// { name: "John", age: 30 }
}).catch((error) => {
console.error(error);
});
How to Implement a Promise based API in JavaScript
When working with asynchronous operations, it’s common to interact with APIs that return promises. You can create your own promise-based APIs to encapsulate asynchronous tasks and provide a cleaner interface for consumers. Here’s an example of implementing a simple promise-based API:
function fetchData() {
return new Promise((resolve, reject) => {
// Simulating an asynchronous operation
setTimeout(() => {
let data = { name: "Alice", age: 25 };
resolve(data); // Resolving the promise with data
}, 2000);
});
}
// Using the promise-based API
fetchData().then((data) => {
console.log(data); // { name: "Alice", age: 25 }
}).catch((error) => {
console.error(error);
});
Introducing Workers in JavaScript
Web Workers are a powerful feature of modern browsers that allow you to run JavaScript code in background threads, separate from the main execution thread. This enables you to perform CPU-intensive tasks without blocking the UI and improves the responsiveness of web applications. Here’s a basic example of using a web worker:
// Creating a new web worker
let worker = new Worker("worker.js");
// Listening for messages from the worker
worker.onmessage = function(event) {
console.log("Message from worker:", event.data);
};
// Sending a message to the worker
worker.postMessage("Hello from main thread!");
5. Client side Web APIs in JavaScript
As you advance, you’ll encounter APIs (Application Programming Interfaces) frequently. They allow you to interact with the browser and access external data. Client-side Web APIs provide interfaces for interacting with various aspects of the web browser and the environment in which your web application runs. Let’s explore some common Client-side Web APIs:
What are APIs in JavaScript ?
APIs provide interfaces for manipulating different aspects of the browser, operating system, or external services. For example, the DOM (Document Object Model) API allows you to manipulate HTML elements.
Introduction to Web APIs in JavaScript
Web APIs, or Application Programming Interfaces, are sets of rules and protocols that allow different software applications to communicate with each other. In the context of web development, Web APIs provide interfaces for manipulating web pages, fetching data from servers, accessing device capabilities, and much more.
Manipulating Documents in JavaScript
The Document Object Model (DOM) API allows you to manipulate the structure and content of HTML documents dynamically. You can use DOM methods and properties to add, remove, or modify elements, change styles, and handle events. Here’s a simple example:
// Accessing an element by its ID
let element = document.getElementById("myElement");
// Changing its text content
element.textContent = "Hello, World!";
Fetching Data from the Server in JavaScript
The Fetch API provides a modern, Promise-based interface for making HTTP requests and fetching resources from servers. It allows you to handle responses asynchronously and provides more flexibility and control over network requests compared to older techniques like XMLHttpRequest. Here’s how you can use the Fetch API to fetch data from a server:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Third party APIs in JavaScript
Third-party APIs allow you to integrate data and functionality from external services into your web applications. These APIs provide access to a wide range of services, including social media platforms, payment gateways, mapping services, and more. Popular examples include the Twitter API, Google Maps API, and PayPal API.
Drawing Graphics in JavaScript
The Canvas API enables you to draw graphics, animations, and interactive content dynamically on a web page using JavaScript. It provides a pixel-based drawing surface and a set of APIs for drawing shapes, paths, text, and images. Here’s a basic example of drawing a rectangle on a canvas:
let canvas = document.getElementById('myCanvas');
let ctx = canvas.getContext('2d');
ctx.fillStyle = 'blue';
ctx.fillRect(10, 10, 100, 100);
Video and Audio APIs in JavaScript
The HTML5 Video and Audio APIs allow you to embed and control multimedia content (such as video and audio files) directly in web pages without requiring third-party plugins like Flash. These APIs provide methods and events for loading, playing, pausing, seeking, and controlling playback of media files.
Client side Storage in JavaScript
Client-side storage APIs, such as Web Storage (localStorage and sessionStorage) and IndexedDB, allow you to store data locally on the user’s device. This data persists even after the user closes the browser or navigates away from the page, providing a convenient way to cache data, store user preferences, and build offline web applications.
This guide provides a solid foundation for your journey into JavaScript. Each module builds upon the previous one, ensuring you grasp the essentials and gain confidence in your programming skills. You’ve covered various topics, from the basics of JavaScript syntax to advanced concepts like asynchronous programming and working with APIs. Practice is key to mastering any programming language, so keep experimenting and building projects. With your newfound knowledge, you’re on your way to becoming a proficient JavaScript developer.