The Ultimate Frontend Developer's Path
Welcome to the dynamic world of front end development, where creativity meets functionality to shape the digital landscape we interact with every day. If you’re just embarking on your journey into front-end development or looking to enhance your skills, you’re in the right place. In this comprehensive roadmap, we’ll guide you through the essential components, tools, and frameworks that will empower you to build captivating and responsive user interfaces.
Table of Contents
1. Understanding Frontend Development
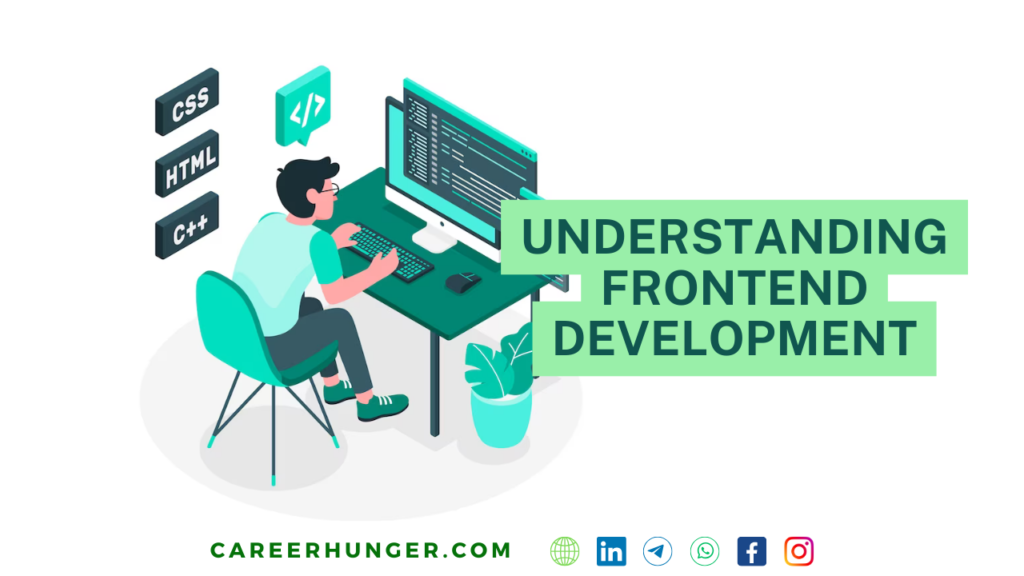
Frontend development is the practice of crafting the user interface and user experience of a website or application. It involves creating visually appealing and interactive elements that users interact with directly. A front-end developer uses languages like HTML, CSS, and JavaScript to translate design concepts into a seamless and engaging digital experience.
2. Learning from Scratch
Frontend development is the art of bringing web designs to life. As you embark on this journey, it’s natural to wonder, “How long does it take to learn everything?” The truth is, that frontend development is a continuous learning process, and the time it takes varies based on your commitment and prior experience. However, this roadmap is designed to streamline your learning process and provide a structured approach.
How Does the Internet Work?
Understanding the basics of how the internet works is crucial for any front-end developer. Explore the concept of interconnected networks, data transmission, and the role of Internet Service Providers (ISPs) in facilitating communication between devices globally.
What is HTTP?
Hypertext Transfer Protocol (HTTP) is the foundation of data communication on the World Wide Web. Learn how HTTP enables the transfer of information between a web server and a web browser, facilitating the retrieval and display of web content.
What is a Domain Name?
A domain name is the human-readable address that users type into their browsers to access a website. Delve into the domain name system (DNS) and understand how domain names are translated into IP addresses, directing users to the correct web server.
What is Hosting?
Hosting involves storing and managing website files on a server to make them accessible on the internet. Explore different hosting options, including shared hosting, virtual private servers (VPS), and cloud hosting, and understand their implications for website performance.
DNS and How it Works
The Domain Name System (DNS) acts as a translator, converting human-readable domain names into IP addresses that machines understand. Learn about the DNS hierarchy, authoritative servers, and the resolution process that enables users to access websites using domain names.
Browsers and How They Work
Browsers are the gateways to the internet, translating HTML, CSS, and JavaScript into visual elements on the screen. Understand the rendering engine, browser components, and the Document Object Model (DOM) that browsers use to display web content.
3. HTML: The Foundation of Web Development
HyperText Markup Language (HTML) serves as the backbone of web development. Start by learning the fundamentals of HTML, and understanding how to structure content on a webpage using tags such as headings, paragraphs, images, and links.
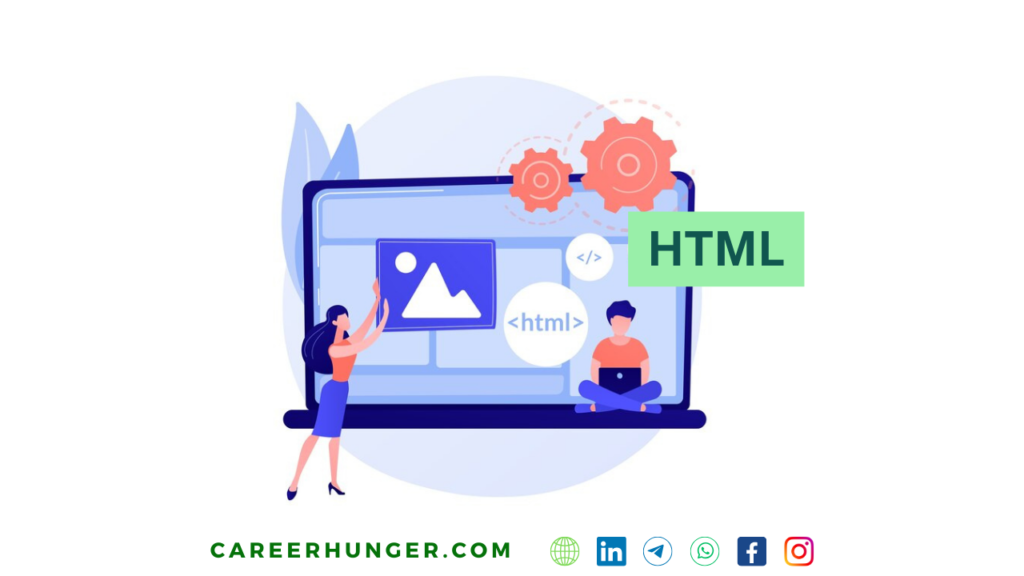
Writing Semantic HTML
Dive into the concept of semantic HTML, where the choice of tags reflects the meaning and structure of the content. Utilize tags like <article>, <section>, and <nav> to enhance both the readability for developers and accessibility for users.
Forms and Validations
Explore the intricacies of HTML forms to gather user input. Learn how to create various form elements such as text inputs, radio buttons, and dropdowns. Understand the importance of client-side form validation to enhance user experience and reduce server load.
Web Accessibility
Delve into the world of web accessibility (a11y) by learning how to make your HTML more inclusive. Understand the importance of ARIA roles, landmarks, and labels to ensure that your web applications are accessible to everyone, regardless of their abilities.
4. CSS: Styling the Web
CSS, or Cascading Style Sheets, is the language that breathes life into your HTML structures. Start by learning the basics of CSS to style and layout your web pages. Understand how to select elements, apply styles, and create visually appealing designs.
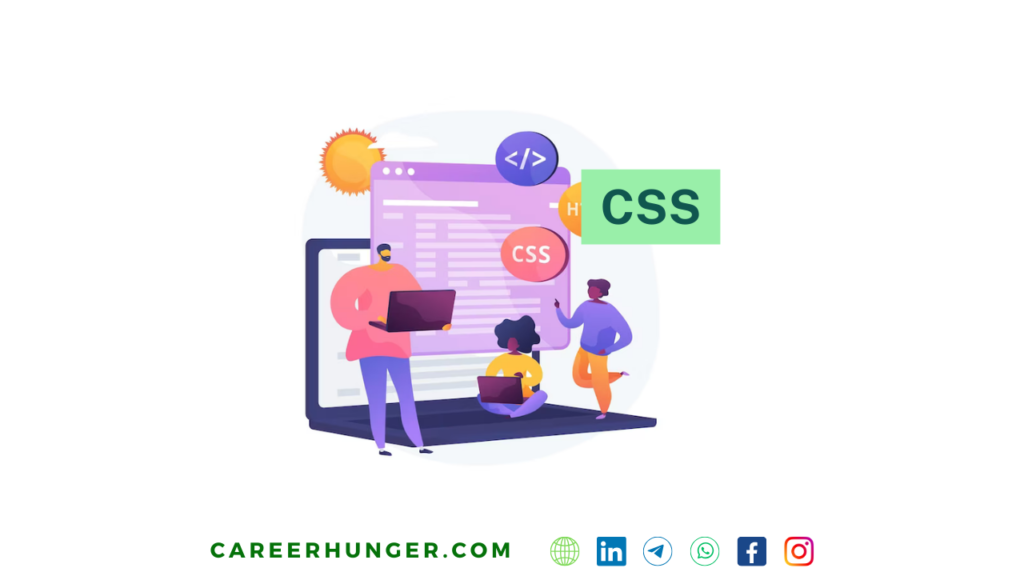
Creating Layouts and Responsive Designs
Delve into the art of crafting layouts using CSS. Learn the principles of flexbox and grid to create responsive and adaptive designs that look great on various devices and screen sizes.
CSS Writing: Tailwind, Radix UI, Shadow CSS
Tailwind CSS: Utilitarian Elegance
Tailwind CSS is a utility-first CSS framework that streamlines the styling process. Discover the power of utility classes to design your interfaces efficiently, speeding up your development workflow without sacrificing flexibility.
Radix UI: Building Consistent Components
Explore Radix UI as a library for building high-quality, accessible components. Radix UI provides a set of primitives that make it easier to create consistent and customizable user interfaces.
Shadow CSS: Elegant Styling
Dive into the world of Shadow CSS, a technique that encapsulates styles within a component, preventing them from affecting the rest of the application. Explore how Shadow CSS can enhance the maintainability and modularity of your styles.
CSS Architecture: BEM (Block Element Modifier)
BEM is a methodology for structuring and naming your CSS classes in a way that promotes maintainability and reusability. Learn how to create a clear and organized CSS architecture by adopting BEM principles.
CSS Preprocessors
CSS preprocessors like Sass and Less extend the capabilities of traditional CSS by introducing variables, nesting, and functions. Explore how preprocessors can enhance your CSS workflow and make styling more efficient.
5. JavaScript: Adding Interactivity
JavaScript is the powerhouse that adds interactivity to your web pages. Begin by learning the basics of JavaScript, including variables, data types, operators, and control flow. Understand how to write functions and work with arrays and objects.
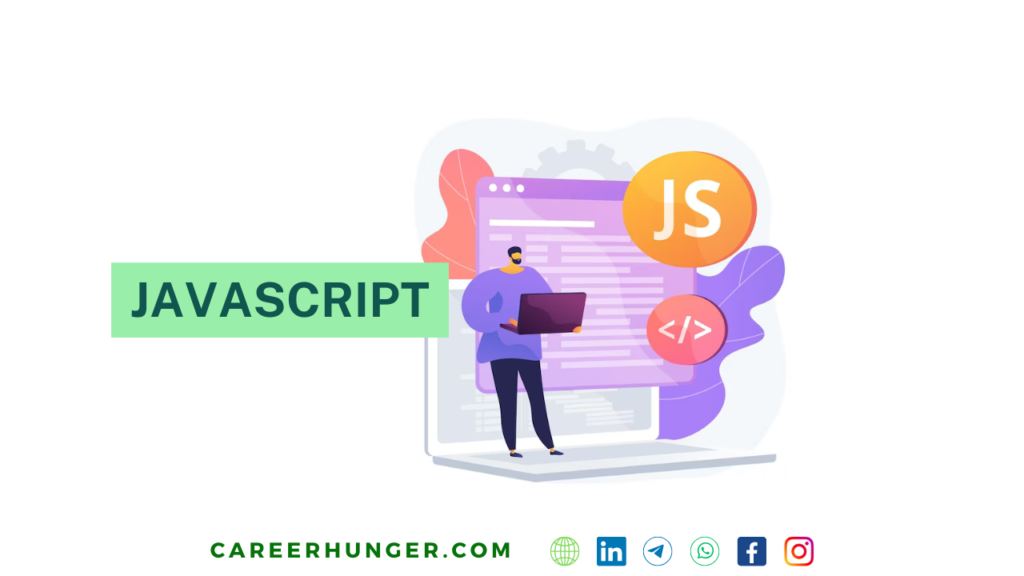
DOM Manipulation
Unlock the true potential of JavaScript by mastering Document Object Model (DOM) manipulation. Learn how to dynamically update and modify HTML and CSS, making your web pages come alive with user interactions.
Fetch API/Ajax(XHR)
Delve into asynchronous programming with JavaScript by exploring Fetch API and Ajax (XMLHttpRequest). Learn how to make HTTP requests to external APIs, enabling your applications to retrieve and send data without refreshing the entire page.
6. Version Control: Tracking Changes
Version control is crucial for managing your codebase. Get familiar with Version Control Systems (VCS) like Git, and understand the importance of tracking changes, collaborating with others, and reverting to previous states.
7. VCS Hosting: A Safe Haven for Your Code
Explore platforms like GitHub, Bitbucket, or GitLab to host your repositories. These platforms provide a collaborative environment, allowing developers to collaborate seamlessly and contribute to open-source projects.
8. Package Manager: Streamlining Dependencies
Package managers simplify the process of managing and installing dependencies for your projects. They automate the retrieval, configuration, and installation of third-party libraries and tools.
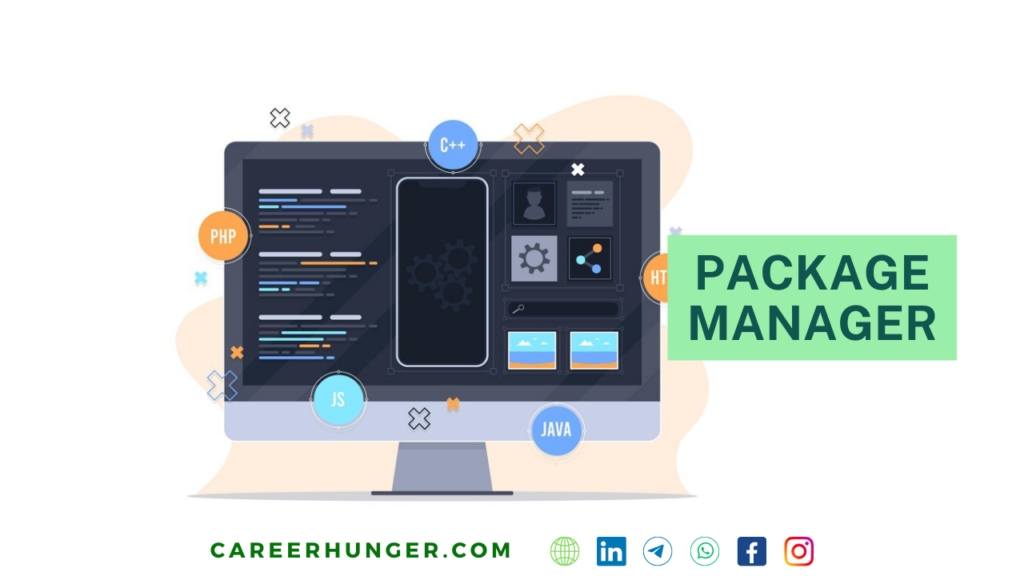
npm: Node Package Manager
npm is the default package manager for the JavaScript runtime environment Node.js. It allows developers to share and reuse code easily. Learn how to use npm to install packages, manage dependencies, and run scripts.
pnpm: Fast, disk space-efficient package manager
pnpm is an alternative package manager that aims to provide a faster and more disk space-efficient alternative to npm. It achieves this by using a single global package store shared across projects. Explore the benefits of pnpm and how it streamlines package management.
Yarn: Fast, reliable, and secure dependency management
Yarn is a package manager developed by Facebook, Google, Exponent, and Tilde. It focuses on speed, reliability, and security. Learn how to use Yarn to manage project dependencies, improve installation times, and lock down dependencies for consistency.
9. Pick a Framework: Building on Solid Foundations
Choosing a frontend framework is a pivotal decision that can significantly impact your development workflow and the performance of your applications. Each framework has its strengths, and the choice depends on factors such as project requirements, personal preferences, and community support.
React: Declarative, Component-Based UI
React, developed by Facebook, is a powerful JavaScript library for building user interfaces. Embrace its declarative syntax and component-based architecture to create reusable, modular UI components. React is widely used and has a robust ecosystem of tools and libraries.
Vue.js: Approachable and Flexible
Vue.js is a progressive JavaScript framework focusing on simplicity and ease of integration. With its approachable learning curve, Vue.js is an excellent choice for both beginners and experienced developers. Learn about Vue’s reactivity system and component-based architecture.
Angular: Full-Fledged MVC Framework
Angular, maintained by Google, is a comprehensive frontend framework that follows the Model-View-Controller (MVC) architecture. It provides a complete solution for building large-scale, enterprise-grade applications. Explore Angular’s two-way data binding, dependency injection, and powerful CLI for efficient development.
Svelte: Cybernetically Enhanced Web Apps
Svelte is a radical approach to building user interfaces. It shifts the work from the browser to the build step, resulting in highly optimized and efficient code. Explore Svelte’s simplicity and performance advantages that make it stand out in the frontend landscape.
SolidJS: Reactive UI Library
SolidJS is a declarative JavaScript library for building user interfaces. With a focus on reactivity, SolidJS enables developers to create efficient and responsive applications. Learn about SolidJS’s reactive primitives and how they enhance the development experience.
Qwik: Reactive State-Driven Framework
Qwik is a reactive state-driven framework that optimizes frontend performance by minimizing the amount of JavaScript shipped to the browser. Explore Qwik’s architecture, designed to provide a fast and efficient user experience while maintaining simplicity in development.
10. Build Tools: Streamlining Your Workflow
Build tools are essential for optimizing and automating various tasks in the front-end development process. These tools help enhance code quality, improve performance, and ensure a smooth development experience.
Module Bundlers
Vite: A Next-Generation Bundler
Vite is a front-end build tool that focuses on speed and simplicity. With a unique approach that leverages ES module imports, Vite delivers fast build times and efficient development workflows. Explore how Vite can revolutionize your front-end development process.
esbuild: Fast, Parallel JavaScript Bundler
esbuild is an ultra-fast JavaScript bundler that prioritizes speed and efficiency. Learn how esbuild leverages parallel processing to deliver rapid build times, making it an excellent choice for modern front-end development.
Webpack: The Versatile Module Bundler
Webpack is a widely used module bundler with extensive plugin support. Dive into Webpack’s capabilities for bundling JavaScript, CSS, and assets, as well as handling complex dependency graphs. Explore how Webpack streamlines the deployment of web applications.
Rollup: JavaScript Module Bundler
Rollup is a JavaScript module bundler that excels at creating optimized bundles for libraries and applications. Understand Rollup’s tree-shaking capabilities, enabling the removal of unused code to create smaller, more efficient bundles.
Parcel: Blazing-Fast, Zero-Configuration Bundler
Parcel is a zero-configuration bundler that simplifies the front-end development process. Explore how Parcel’s automatic setup and fast build times make it an excellent choice for small to medium-sized projects.
Task Runners
npm Scripts: Automating Tasks with JavaScript
npm Scripts is a powerful way to automate various tasks in your front-end development workflow. Learn how to leverage npm Scripts to run build processes, execute tests, and perform other custom tasks, enhancing your project’s automation.
Linters and Formatters
Linters and formatters play a crucial role in maintaining code quality and consistency within a project. Explore the importance of linting for identifying and fixing potential issues in your code. Learn how formatters ensure consistent code styling, enhancing collaboration among developers.
11. Testing Your Apps: Ensuring Reliability
Testing is an integral part of the development process, ensuring that your applications are reliable, functional, and bug-free. Explore different testing frameworks and tools to enhance the quality of your code.
Vitest: Minimalist Testing Framework
Vitest is a minimalist testing framework designed for simplicity and efficiency. Discover how Vitest can help you write clean and concise tests, making it easier to ensure the reliability of your applications.
Jest: Delightful JavaScript Testing
Jest is a popular testing framework focusing on simplicity and ease of use. Learn how Jest provides a delightful testing experience, including features like snapshot testing, mocking, and parallel test execution.
Playwright: End-to-end Testing
Playwright is a powerful end-to-end testing framework that enables you to automate browser interactions. Explore how Playwright allows you to simulate user actions, test complex scenarios, and ensure the functionality of your applications across different browsers.
Cypress: Fast, Easy, and Reliable Testing
Cypress is a fast and reliable end-to-end testing framework that provides a real-time preview of your application as tests run. Learn how Cypress simplifies the testing process, allowing you to write and execute tests with ease.
12. Authentication Strategies: Securing User Data
User authentication is a critical aspect of web development. Delve into various authentication strategies, including OAuth, JWT, and sessions, to ensure your applications are secure and user-friendly.
Basic Authentication
Understand the concept of Basic Authentication, where the user’s credentials (username and password) are transmitted as a base64-encoded string. Learn about its simplicity and potential security concerns.
Session-Based Authentication
Explore session-based authentication, where a server generates a unique session identifier for each user upon successful login. Understand how sessions help maintain user states throughout their interaction with the application.
Token-Based Authentication
Delve into token-based authentication, where a token is issued to users upon successful login and is used for subsequent requests. Learn about the advantages of token-based systems in enhancing security and scalability.
JWT Authentication
Explore JSON Web Token (JWT) authentication, a compact, URL-safe means of representing claims to be transferred between two parties. Learn how JWTs can be used to transmit information between the client and server securely.
OAuth
Dive into OAuth, an open standard for access delegation commonly used as a way for Internet users to grant third-party websites or applications access to their information without sharing their credentials. Explore its use in enabling secure authorization.
SSO (Single Sign-On)
Understand the concept of Single Sign-On, where a user can access multiple applications with a single set of credentials. Learn how SSO enhances user experience and simplifies authentication across various platforms.
13. Server-Side Rendering: Enhancing Performance
Server-side rendering (SSR) is a technique that involves rendering a web page on the server rather than the client’s browser. This approach enhances performance and provides benefits like improved search engine optimization (SEO) and faster initial page loads.
React
Next.js: Unleashing React's SSR Power
Next.js is a React framework that enables server-side rendering, making React applications faster and more SEO-friendly. Explore how Next.js simplifies the process of implementing SSR in React applications, enhancing performance and user experience.
Remix: Blending React with Server-Side Rendering
Remix is a full-stack React framework that emphasizes server-rendered pages and efficient data fetching. Learn how Remix provides a delightful development experience by seamlessly integrating server-side rendering into React applications.
Angular
Angular Universal: Server-Side Rendering for Angular
Angular Universal extends the Angular framework to enable server-side rendering. Discover how Angular Universal improves performance by rendering Angular applications on the server, delivering faster initial page loads.
Vue.js
Nuxt.js: SSR Magic for Vue.js
Nuxt.js is a framework for Vue.js that brings server-side rendering to Vue applications. Explore how Nuxt.js simplifies the implementation of SSR, providing benefits like improved SEO and faster page loads.
Svelte
Svelte Kit: SSR for Cybernetically Enhanced Web Apps
Svelte Kit extends Svelte’s capabilities to include server-side rendering, resulting in highly optimized web applications. Learn how Svelte Kit streamlines the development of SSR-enabled applications, combining the best of Svelte and SSR.
14. GraphQL: Efficient Data Query Language
GraphQL is a query language for APIs that provides a more efficient and powerful alternative to traditional REST APIs. It allows clients to request only the data they need, reducing over-fetching and under-fetching of data. Let’s explore the fundamentals of GraphQL and its integration into front-end development.
Apollo: Data Management Made Easy
Apollo Client:
Apollo Client is a fully-featured, community-driven GraphQL client for React, Vue, and Angular. It simplifies data fetching and state management by seamlessly integrating with your favorite front-end frameworks.
Apollo Server:
Apollo Server, paired with Apollo Client, enables you to build a comprehensive GraphQL server. It allows you to define your data graph and efficiently handle data queries and mutations.
Relay Modern: Declarative Data Fetching
Modern Approach:
Relay Modern is a GraphQL client developed by Facebook, designed to work seamlessly with React. It adopts a declarative approach to data fetching, allowing developers to specify the data requirements of their components.
Automatic Batching and Caching:
Relay Modern provides automatic batching and caching of GraphQL queries, optimizing network requests and enhancing the performance of your applications.
GraphQL, Apollo, and Relay Modern together offer a powerful combination for efficient data management in modern front-end development. Understanding these technologies will empower you to build scalable and performant applications. Happy coding!
15. Static Site Generators: Blazing-Fast Websites
Static Site Generators (SSGs) transform your content into HTML files during the build process, resulting in fast, secure, and easily deployable websites. Let’s explore some popular SSGs and how they can elevate your web development projects.
Astro: Faster Rendering, Smarter Bundling
Zero-Render Philosophy:
Astro adopts a zero-render philosophy, delivering the minimum amount of JavaScript needed for interactivity. This results in faster page loads and a smoother user experience.
Smart Bundling:
Astro’s smart bundling optimizes the delivery of JavaScript and CSS, reducing the payload size and enhancing website performance.
Eleventy: Simplicity and Flexibility
Simplified Configuration:
Eleventy stands out for its simplicity. With minimal configuration, it allows you to focus on creating content without unnecessary complexities.
Flexibility:
Eleventy supports various template engines, giving you the flexibility to choose the one that best fits your preferences and project requirements.
Next.js: Versatile React Framework
Static Site Generation:
Next.js offers static site generation, enabling you to pre-render pages at build time for lightning-fast performance.
Hybrid Approach:
Next.js supports both static site generation and server-side rendering, providing a hybrid approach for dynamic content when needed.
Remix: Full-Stack React Framework
Data Fetching Strategies:
Remix emphasizes efficient data fetching strategies, enabling you to fetch data at the edge and pre-render pages with minimal JavaScript in the client.
Dynamic Loading:
Remix supports dynamic loading, allowing you to fetch data on the client side as users navigate through your application.
Vuepress: Vue.js-Powered Documentation
Built for Documentation:
Vuepress is designed specifically for documentation projects, providing an easy-to-use structure for creating and maintaining documentation websites.
Vue-powered Theming:
With Vue.js at its core, Vuepress allows you to leverage Vue-powered theming, making it seamless to customize the appearance of your documentation.
Jekyll: Simple Blogging and Static Sites
Ruby-Powered:
Jekyll, powered by Ruby, is a simple and blog-aware static site generator. It’s well-suited for personal websites, blogs, and portfolios.
GitHub Pages Integration:
Jekyll integrates seamlessly with GitHub Pages, making it easy to host your static sites directly from your GitHub repository.
Hugo: Speedy Go-Powered Generator
Lightning-Fast Builds:
Hugo is known for its blazing-fast build times, making it an excellent choice for large-scale static sites or blogs.
Go Language Efficiency:
Built with Go, Hugo ensures efficiency in generating static content, resulting in rapid development cycles.
Nuxt.js: Vue.js-Powered Static Sites
Versatile Framework:
Nuxt.js extends Vue.js to create powerful static sites with its built-in support for static site generation.
Vue Components:
Leverage Vue components and the Vue ecosystem to build static sites with dynamic and interactive elements.
Static Site Generators offer a range of options to suit different project requirements. Choose the one that aligns with your preferences and project needs for optimal results.
16. Mobile Applications: Extending Your Reach
In a mobile-first world, creating cross-platform mobile applications has become essential. Let’s explore some popular frameworks that enable front-end developers to extend their skills into the realm of mobile app development.
React Native: Cross-Platform React
JavaScript Power:
React Native allows you to use your JavaScript skills to build cross-platform mobile applications that feel native on both iOS and Android.
Reusable Components:
Leverage React components to create reusable UI elements and logic, streamlining development for multiple platforms.
Flutter: Beautiful, Fast, Native Apps
Dart Language:
Flutter uses Dart, a language optimized for building mobile, desktop, server, and web applications, providing a cohesive and productive development experience.
Widget-Based UI:
Flutter’s UI is entirely widget-based, allowing you to create a consistent and visually appealing user interface across platforms.
Ionic: Cross-Platform Hybrid Apps
Web Technologies:
Ionic enables you to use web technologies such as HTML, CSS, and JavaScript to build cross-platform hybrid mobile applications.
Angular Integration:
Integrated with Angular, Ionic provides a robust framework for building high-quality mobile applications with a single codebase.
NativeScript: Truly Native Mobile Apps
NativeScript Core:
NativeScript allows you to build native mobile apps using JavaScript or TypeScript. With NativeScript Core, you can access native APIs directly.
Angular or Vue Integration:
Integrate with Angular or Vue.js for a familiar component-based development experience while maintaining native performance.
17. Desktop Applications in JavaScript: Beyond the Browser
JavaScript has evolved beyond web browsers, and developers can now build powerful desktop applications using familiar technologies. Let’s explore some frameworks that empower front-end developers to create cross-platform desktop applications.
Electron: Cross-Platform Desktop Apps
Chromium and Node.js:
Electron leverages Chromium for rendering web pages and Node.js for backend functionality, enabling the development of cross-platform desktop applications.
Web Technologies:
Build desktop apps using web technologies such as HTML, CSS, and JavaScript. Electron allows you to reuse your web development skills for desktop applications.
Tauri: Lightweight and Fast
Rust and JavaScript:
Tauri takes a unique approach by using Rust for the backend and JavaScript for the front end. This combination results in lightweight, fast, and secure desktop applications.
No External Runtime:
Tauri doesn’t rely on an external runtime like Node.js, contributing to smaller app sizes and improved performance.
Flutter for Desktop: Consistent Multi-Platform UIs
Single Codebase:
Flutter extends its capabilities to desktop platforms, allowing you to maintain a single codebase for mobile, web, and desktop applications.
High-Quality UI:
Flutter’s widget-based UI system ensures a consistent and high-quality user interface across various platforms, including desktops.
Building desktop applications in JavaScript offers a versatile and efficient approach. Explore these frameworks to create powerful and user-friendly desktop experiences using your existing front-end development skills. Happy coding!
Congratulations! You’ve traversed the vast landscape of front-end development, from laying the HTML foundation to building dynamic interfaces with JavaScript and styling with CSS. Remember, the key to success lies in acquiring knowledge and applying it through hands-on projects. This roadmap is your compass, guiding you through the exciting journey of front-end development. Keep coding, stay curious, and let your creativity shape the digital experiences of tomorrow. Happy coding!